Sometimes it makes sense to preselect answer options when Respondent passes the survey.
For example, you may include a hidden question into Questionnaire for saving some additional information like the order of selected alternatives in question, or display a summary of Respondent’s answers at the end of survey.
In the example below we will demonstrate how to create a summary page and present it to Respondent. We will also show how to prevent Respondent from changing the summary page (the data will be displayed in “read only” mode).
Try how it works below
In order to reproduce this example, please undertake the following steps:
Create two “Single Answer” questions and one "Multiple Answers" question. The last one will display the summary of answers received in first two questions. Use the text of the first question as an alternative in the last one (i.e. insert the question text into first “Answer options” textbox) and enable the “Subheader” checkbox (as shown in the image below).
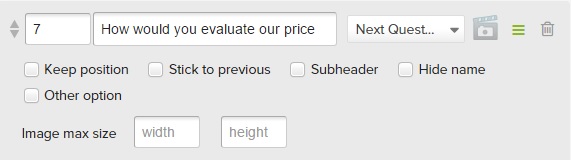
Create the same alternatives as in the first question (don’t pay attention to their codes, only the text matters).
Repeat the abovementioned actions, but this time use the data from the second question: first, text of the question (don’t forget about "Subheader" checkbox), and then text of the alternatives.
After all, launch the Script Editor (in the third question) and insert the following script into "OnRender script" tab (see the image below). Take note of the 'set()' function in our script. It selects alternative in the question.
|
![]() |
After that modify the tokens highlighted in red as described in the table below:
FirstCode | Code of the first question |
SecondCode | Code of the second question |
codeFAnswer | Code of the alternative which has been selected as answer in the first question |
codeSAnswer | Code of the alternative which has been selected as answer in the second question |
q1alt1 | Code of the first alternative in the first question |
q1alt2 | Code of the second alternative in the first question |
q1alt3 | Code of the third alternative in the first question |
q1alt4 | Code of the fourth alternative in the first question |
q1alt5 | Code of the fifth alternative in the first question |
q2alt1 | Code of the first alternative in the second question |
q2alt2 | Code of the second alternative in the second question |
q2alt3 | Code of the third alternative in the second question |
q2alt4 | Code of the fourth alternative in the second question |
q2alt5 | Code of the fifth alternative in the second question |
q3alt2 | Code of the second alternative in the third question |
q3alt3 | Code of the third alternative in the third question |
q3alt4 | Code of the fourth alternative in the third question |
q3alt5 | Code of the fifth alternative in the third question |
q3alt6 | Code of the sixth alternative in the third question |
q3alt8 | Code of the eighth alternative in the third question |
q3alt9 | Code of the ninth alternative in the third question |
q3alt10 | Code of the tenth alternative in the third question |
q3alt11 | Code of the eleventh alternative in the third question |
q3alt12 | Code of the twelfth alternative in the third question |
Have you noticed that the first and the sixth alternatives of the third question are missing in our table? We didn’t include them, because those alternatives are the subheaders, and we are not interested in their codes.
Advanced explanations
- var codeFAnswer = q['FirstCode'].get()[0].code;
- // get the code of alternative which has been selected as an answer in the first question and assign it to 'codeFAnswer' Since the first question has "Single Answer" type, it always contains only one alternative in the array of answers. So, we can get the code explicitly by using '[0]' pointer
- var codeSAnswer = q['SecondCode'].get()[0].code;
- // get the code of alternative which has been selected as an answer in the second question and assign it to 'SecondCode'. Here we also get the answer explicitly by using '[0]' pointer.
- switch (codeFAnswer ) {
- // evaluate the code of the first answer
- case 'q1alt1':
- this.set('q3alt2', true);
- //and if it equals the code of the first alternative in the first question, then we chose second alternative in the third question
- break;
- case 'q1alt2':
- this.set('q3alt3', true);
- break;
- case 'q1alt3':
- this.set('q3alt4', true);
- break;
- case 'q1alt4':
- this.set('q3alt5', true);
- break;
- case 'q1alt5':
- this.set('q3alt6', true);
- break;
- }
- switch (codeSAnswer ) {
- case 'q2alt1':
- this.set('q3alt8', true);
- break;
- case 'q2alt2':
- this.set('q3alt9', true);
- break;
- case 'q2alt3':
- this.set('q3alt10', true);
- break;
- case 'q2alt4':
- this.set('q3alt11', true);
- break;
- case 'q2alt5':
- this.set('q3alt12', true);
- break;
- }
- // this part of the script disables changing of the chosen alternatives
- $('.survey-alternative-line').each( function( ) {
- $(this).off("click");
- });